Keywords in Python
We know how each word in our language has some meaning and when used with the correct grammar, we communicate. Same way, we use keywords and syntax to communicate with the machine.
Python Keywords are predefined reserved words used in Python codes. These are also known as Python-reserved words. These have special meanings.
Keywords are used to define the syntax of the coding. The way our language is made up of grammar and words to communicate something meaningful to other people. In the same way, the programming language is made up of syntax and keywords.
Sounds too technical? Wait, let’s get there with an easy example!
Let’s understand the functioning of keywords with an easy example!
So how do we instruct traffic lights in our language?
“if the signal is green, then proceed, else, stop.”
So if we look at the above statement, we use the grammar and words in a proper way so it makes sense. Same way, we use keyword in Python to make sense for machines.
If I instruct someone without a proper structure of keywords and grammer, say-
“green is if the else proceed signal, stop then”
Worth crashing into a vehicle, isn’t it? This is why we have to use correct syntax in language.
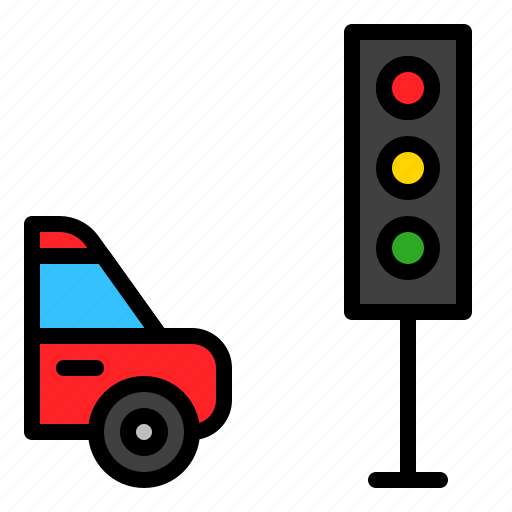
The way we communicate to a human is
“if the signal is green, then proceed else, stop.”
And if we instruct the same statement to machine in programming, this will loosely look like this:
"if signal is green, print 'proceed' else, print 'stop'"
Rearranging for the machine to understand a Python code looks like this-
if signal is green : print("Proceed") else : print("Stop")
The proper code for this looks like this-
signal = "Green" # ← This indicates an actual signal which is green if signal is "Green" : # ← Checking the condition if the signal is green print("Output:"," Proceed" # ←Tells computer what to do if condition in "if keyword" is true. else : # ← Telling computer what to do with "else keyword" if the previous condition is not matching print("Output:"," Stop") """ Output: Proceed """
signal = "Red" if signal is "Green" : #if keyword is being used to tell computer about particular a condition. print("Output:"," Proceed") else : #else keyword is used here to tell computer about another condition. print("Output:"," Stop") """ output: Output: Stop """
In above code, if and else are keywords. When you write keywords, these words have a specific color, like we can see when we wrote if and else. You can check the color in your Python code editor.
All keywords of Python will have same color.
Other things like signal and print are statements. Now, what we said previously “Keywords are used to define the syntax of the coding” must be making some sense.
Let’s have a quick look over some other keywords.
True # True keyword is used to tell computer if a condition is true. False # False keyword is used to tell computer if a condition is false continue # continue keyword is used to tell computer to continue break # break keyword is used to tell computer to break out of a condition if # if keyword is used to tell about a condition (it will match if the condition is true). else # else keyword is used with if, to tell computer what else to do if the previous condition is not satisfied. # The keywords function almost like we use these words in english. It is not hard to get an idea of what a keyword does.
print("will", "this", end= , sep=) """ output: File "<ipython-input-1-0711b6e3925b>", line 1 print("will", "this", end= , sep=) ^ SyntaxError: invalid syntax """
We can understand more about these when we try each one on our own. We will practice with these in further blogs.
Make sure to know these keywords correctly, as we will use them forward. Of course, you don’t need to remember those, but you need to take care while using them.
There are total 33 keywords in Python 3.7.
# Important: You can use help function to check all keywords in python. Write help("function") to get list of all keywords. help("keywords") """ output: Here is a list of the Python keywords. Enter any keyword to get more help. False class from or None continue global pass True def if raise and del import return as elif in try assert else is while async except lambda with await finally nonlocal yield break for not """
Have a look at Python keywords and their description:
No. Keywords Description
- “ and “ : This is a logical operator it returns true if both the operands are true else return false.
- “ Or “ : This is also a logical operator it returns true if anyone operand is true else return false.
- “ not “ : This is again a logical operator it returns True if the operand is false else return false.
- “ if “ : This is used to make a conditional statement.
- “ elif “ : Elif is a condition statement used with if statement the elif statement is executed if the previous conditions were not true
- “ else “ : Else is used with if and elif conditional statement the else block is executed if the given condition is not true.
- “ for “ : This is created for a loop.
- “ while “ : This keyword is used to create a while loop.
- “ break “ : This is used to terminate the loop.
- “ as “ : This is used to create an alternative.
- “ def “ : It helps us to define functions.
- “ lambda “ : It used to define the anonymous function.
- “ pass “ : This is a null statement that means it will do nothing.
- “ return “ : It will return a value and exit the function.
- “ True “ : This is a boolean value.
- “ False “ : This is also a boolean value.
- “ try “ : It makes a try-except statement.
- “ with “ : The with keyword is used to simplify exception handling.
- “ assert “ : This function is used for debugging purposes. Usually used to check the correctness of code
- “ class “ : It helps us to define a class.
- “ continue “ : It continues to the next iteration of a loop
- “ del “ : It deletes a reference to an object.
- “ except “ : Used with exceptions, what to do when an exception occurs
- “ finally “ : Finally is use with exceptions, a block of code that will be executed no matter if there is an exception or not.
- “ from “ : The form is used to import specific parts of any module.
- “ global “ : This declares a global variable.
- “ import “ : This is used to import a module.
- “ in “ : It’s used to check if a value is present in a list, tuple, etc, or not.
- “ is “ : This is used to check if the two variables are equal or not.
- “ None “ : This is a special constant used to denote a null value or avoid. It’s important to remember, 0, any empty container(e.g empty list) do not compute to None
- “ nonlocal “ : It’s declared a non-local variable.
- “ raise “ : This raises an exception
- “ yield “ : It’s ends a function and returns a generator.
# You can use library keyword.kwlist, to get a list of keywords supported by the current version of python you are running the code on. import keyword keyword.kwlist """ output: ['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield'] """
We cannot use keyword names as identifier. That is why it was mentioned that these are Python reserved words.
To understand more about this. Please refer our variable blog.
yield = 1 #here we have used "yield" keyword as variable name """ output: File "<ipython-input-2-7c685dc77939>", line 1 yield = 1 ## here we have used ^ SyntaxError: invalid syntax """
Value and Operator Keywords
Example 1: Example of and, or, not, True, False, None keywords.
print("example of True, False, and, or not keywords") # compare two operands using and operator print(True and True) # compare two operands using or operator print(True or False) # use of not operator print(not False) a = 'co-learning lounge' print('learning' in a) print(True is False) """ output: example of True, False, and, or not keywords True True True True False """
Iteration Keywords
Example 2: Example of a for, while, break, and continue.
# execute for loop for i in range(1, 11): # print the value of i print(i) # check the value of i is less then 5 # if i lessthen 5 then continue loop if i < 5: continue # if i greather then 5 then break loop else: break """ output: 1 2 3 4 5 """
Example 3: example of for, in, if, elif and else keyword.
# run for loop for t in range(1, 5): # print one of t ==1 if t == 1: print('One') # print two if t ==2 elif t == 2: print('Two') else: print('else block execute') """ output: One Two else block execute else block execute """
Control Flow Keywords
Example 4: Example of if, elif and else keywords.
# define Paul() function using def keyword def Paul(i): # check i is odd or not # using if and else keyword if(i % 2 == 0): return "given number is even" elif(i % 2 == 1): return "given number is odd" else: return "Default condition" # call Paul() function print(Paul(10)) print(Paul(25)) """ output: given number is even given number is odd """
Exception Handling Keywords
Example 5: example try, except, raise.
def fun(num): try: r = 1/num except: print('Exception raies') return return r print(fun(10)) print(fun(0)) """ output: 0.1 Exception raies None """
Structural Keywords
Example 6: Example of a def, lambda, pass keyword.
# define a anonymous using lambda keyword # this labda function increment the value of b a = lambda b: b+1 # run a for loop for i in range(1, 6): print(a(i)) pass """ output: 2 3 4 5 6 """
Return Keywords
Example 7: use of return keyword.
# define a function def fun(): # declare a variable a = 5 # return the value of a return a # call fun method and store # it's return value in a variable t = fun() # print the value of t print(t) """ output: 5 """
Variable Handling Keywords
Example 8: use of a del keyword.
# create a list l = ['a', 'b', 'c', 'd', 'e'] # print list before using del keyword print(l) del l[2] # print list after using del keyword print(l) """ output: ['a', 'b', 'c', 'd', 'e'] ['a', 'b', 'd', 'e'] """
Example 9: use of global keyword.
# declare a variable gvar = 10 # create a function def fun1(): # print the value of gvar print(gvar) # declare fun2() def fun2(): # declare global value gvar global gvar gvar = 100 # call fun1() fun1() # call fun2() fun2() """ output: 10 """
Example 10: example of assert keyword.
def sumOfMoney(money): assert len(money) != 0,"List is empty." return sum(money) money = [] print("sum of money:",sumOfMoney(money)) """ output: --------------------------------------------------------------------------- AssertionError Traceback (most recent call last) <ipython-input-2-82410893a392> in <module>() 4 5 money = [] ----> 6 print("sum of money:",sumOfMoney(money)) <ipython-input-2-82410893a392> in sumOfMoney(money) 1 def sumOfMoney(money): ----> 2 assert len(money) != 0,"List is empty." 3 return sum(money) 4 5 money = [] AssertionError: List is empty. """
Example 11: example of yield keyword.
def Generator(): for i in range(6): yield i+1 t = Generator() for i in t: print(i) """ output: 1 2 3 4 5 6 """
We have discussed about keywords so far and have gone through different use cases as well. Keywords will be useful for us while learning other important things.
Now we know that keywords for programming are as fundamental as grammer in our languages. Keyword define the syntax of our program hence it is essential part to convert our logics into code.
We are going to know more features of these when we implements these while learning next concepts.