List Functions in Python
We know how powerful list datatype is. Some methods can help us work with Lists faster, and we will explore all of those in the next examples.
These methods can update, delete, sort, and remove elements from the lists.
Let’s first start by adding new elements to the list.
Adding New Elements to a List using append() method
We can add elements in Lists. Using append()
method Elements can be added to the List using the built-in append() function. Only one element at a time can be added to the list by using the append() method. Tuples can also be added to the List using the append method because tuples are immutable. Unlike sets, Lists can also be added to the existing list using the append() method. We will cover Tuples and sets later, don’t worry about these for now.
Let’s try adding new items to our list.
# Python program to demonstrate # Addition of elements in a List # Creating a List List = [5, 6] print("Initial List: ") print(List) # Addition of Elements # in the List List.append(1) print("\nList after Addition of 1st element: ") print(List) List.append(2) print("\nList after Addition of 2nd element: ") print(List) List.append(4) print("\nList after Addition of Three elements: ") print(List) """ output: Initial List: [5, 6] List after the Addition of 1st element: [5, 6, 1] List after the Addition of 2nd element: [5, 6, 1, 2] List after the Addition of Three elements: [5, 6, 1, 2, 4] """
See how we have added different items to the list. The limitation is we can only add one item with the append function; that’s why in previous examples, we have used for four append functions to add four elements. 🙂
Let’s try adding two items at once! 🙂
# What if we want to add more than one item to the list? var1 = 7 var2 = 8 List.append(7, 8) print(List) ## Well, Append only takes 1 argument """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-5-4e27706f6e5c> in <module> 4 var2 = 8 5 ----> 6 List.append(7, 8) 7 print(List) ## Well, Append only takes 1 argument TypeError: append() takes exactly one argument (2 given) """
Let’s try to find out how to tackle this problem! Because clearly, this is not the correct way to add items to the list.
Suppose you have to add 100, 200, or more items; it doesn’t make sense.
Well, we can add values as a list.
Let’s try one example!
# What if we want to add more than one item to the list? List = [5, 6, 1, 2, 4] var1 = 7 var2 = 8 l = [var1, var2] List.append([7, 8]) print(List) ## But adding a list using appends as a list. Not a invidual value. # Adding Tuples to the List List.append((5, 6)) print("\nList after Addition of a Tuple: ") print(List) # Addition of List to a List List2 = ['For', 'learners'] List.append(List2) # Appending through variable "List2" print("\nList after Addition of a List: ") print(List) """ output: [5, 6, 1, 2, 4, [7, 8]] List after Addition of a Tuple: [5, 6, 1, 2, 4, [7, 8], (5, 6)] List after Addition of a List: [5, 6, 1, 2, 4, [7, 8], (5, 6), ['For', 'learners']] """
In the above example, we have added multiple items at once using list append. We can’t add more than 1 item; better that item be another List! 😆
Also, don’t worry about the tuple for now; we are going to cover that later.
To append multiple elements, the loop can be used to iterate and append() one at a time.
Looping is a way to use the same code multiple times until a condition is satisfied. We are going to explore this later. For now, just understand this example; suppose you are listening to say “Perfect by Ed-Sheeran” and you are about to sleep, so you put it on a loop five times because you are about to sleep (the song will play five times and then it will stop), this is what looping is.
You instructed the program that play--perfect for 5 times
, the program would repeat the same code to play the song five times and then close. This is what we do in looping.
You can see the example below!
# Adding elements to the List # using Iterator List = [] for i in range(1, 4): List.append(i) print("\nList after Addition of elements from 1-3: ") print(List) new_list = [4, 5, 6, 7] for j in new_list : List.append(j) print("\nList after Addition of new elements from new list: ") print(List) """ output: List after Addition of elements from 1-3: [1, 2, 3] List after Addition of new elements from new list: [1, 2, 3, 4, 5, 6, 7] """
Using extend() method
If .append() with for loop looks infeasible then .extend() can be used. append reduce our work and add all the elements passed as arguments in the original list.
Note — append() and extend() methods can only add elements at the end.
Let’s try one example!
# Python program to demonstrate # Addition of elements in a List # Creating a List List = [1,2,3,4] print("Initial List: ") print(List) # Addition of multiple elements # to the List at the end # (using Extend Method) List.extend([8, 'co-learners', 'Always']) print("\nList after performing Extend Operation: ") print(List) List.extend((9, 10)) # Typically, list is passed to .extend(), but it could also be a tuple. print("\nList after performing Extend Operation: ") print(List) """ output: Initial List: [1, 2, 3, 4] List after performing Extend Operation: [1, 2, 3, 4, 8, 'co-learners', 'Always'] List after performing Extend Operation: [1, 2, 3, 4, 8, 'co-learners', 'Always', 9, 10] """
Extend also takes only one argument, like append, and iterates over it. The length of the original List is increased by the number of iterations.
Let’s understand this way:
(["Co-Learning Lounge" , "Python", "Interesting"])
— With this argument, the length will decrease by three because three elements are added.
(Python)
— with this, the length will increase by 6.
Let’s see how!
NewList = ["Love", "Learn"] NewList.extend(["Co-Learning Lounge" , "Python", "Interesting"]) print(NewList) #3 items will be added coz it can iterated over thrice. NewList.extend("Python") print(NewList) #It can be iterated over 6 items with each character NewList.extend(["Lists"]) print(NewList) #can only be iterated once so 1 element will be added """ output: ['Love', 'Learn', 'Co-Learning Lounge', 'Python', 'Interesting'] ['Love', 'Learn', 'Co-Learning Lounge', 'Python', 'Interesting', 'P', 'y', 't', 'h', 'o', 'n'] ['Love', 'Learn', 'Co-Learning Lounge', 'Python', 'Interesting', 'P', 'y', 't', 'h', 'o', 'n', 'Lists'] """
List.extend(8, 'co-learners', 'Always') ## Please note extend still except a single value only i.e list print(List) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-4-6de8a5c1511e> in <module> ----> 1 List.extend(8, 'co-learners', 'Always') ## Please note extend still except a single value only i.e list 2 print(List) TypeError: extend() takes exactly one argument (3 given) """
extend() function is basically iteration using the append function, and for iteration, we need values that can be iterated.
Hence if we add only one value that can’t be iterated, extend() won’t work!
List.extend(9) ## Even if you pass a single value which is not iterable it will through errors. list, tuple, dict, set can be iterate over print(List) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-5-8f2e9fe5627c> in <module> ----> 1 List.extend(9) ## Even if you pass a single value which is not iterable it will through errors. list, tuple, dict, set can be iterate over 2 print(List) TypeError: 'int' object is not iterable """
List.extend([90, 100]) print(List) """ output: [1, 2, 3, 4, 8, 'co-learners', 'Always', 9, 10, 9, 90, 100] """
Using insert() method
append() and extend() method only works for adding elements at the end of the List. The insert() method is used to add elements at the desired position.
list.insert(index, element)
- index (Required): A number specifying in which position to insert the value
- element (Required): An element of any type (string, number, object, etc.)
Unlike append() and extend() which takes only one argument, insert() method requires two arguments(position, value).
# Python program to demonstrate # Addition of elements in a List # Creating a List List = [1,2,3,4] print("Initial List: ") print(List) # Addition of Element at # specific Position # (using Insert Method) List.insert(3, 12) print(List) List.insert(0, 'co-learners') print("\nList after performing Insert Operation: ") print(List) print("\nLength of the list is: ", len(List)) List.insert(10, 120) ## Elements can be added at any index. If that index is out of range which is in this case. # New length of the 6 but we are adding new element on 10th index. It will by default put it at the end print(List) List.insert(-2, 78.18) print("\nList after adding in the second last place: ") print(List) """ output: Initial List: [1, 2, 3, 4] [1, 2, 3, 12, 4] List after performing Insert Operation: ['co-learners', 1, 2, 3, 12, 4] Length of the list is: 6 ['co-learners', 1, 2, 3, 12, 4, 120] List after adding in the second last place: ['co-learners', 1, 2, 3, 12, 78.18, 4, 120] """
Sort and Reverse methods
Syntax of sort() is,
list.sort(key=..., reverse=...)
sort() doesn’t need any default parameters. Although, it has two optional parameters:
- reverse — If True, the sorted list is reversed (or sorted in Descending order)
- key — function that serves as a key for the sort comparison
List = [6, 1, 7, 1, 3, 9, 2, 8, 4, 10, 27, 219] List.sort() print(List) List.sort(reverse=True) print("\nSorted in Descending order") print(List) """ output: [1, 1, 2, 3, 4, 6, 7, 8, 9, 10, 27, 219] Sorted in Descending order [219, 27, 10, 9, 8, 7, 6, 4, 3, 2, 1, 1] """
def get_second_value(item): return item[1] items = [(10, 3), (-9, 1), (5, -1), (9, 2)] items.sort(key=get_second_value) print(items) """ output: [(5, -1), (-9, 1), (9, 2), (10, 3)] """
We can also use the reverse function directly.
List = [219, 27, 10, 9, 8, 7, 6, 4, 3, 2, 1, 1] List.reverse() print(List) """ output: [1, 1, 2, 3, 4, 6, 7, 8, 9, 10, 27, 219] """
Copying the list with copy() function
We can make a copy of the original list by using copy() function. Let’s try!
name = ["co-learning", "lounge", "is", "the", "best", "community"] name_1 = name.copy() name_2 = name ## Print values after poping the value name.pop() print(name) print("id of the community variable ", id(name), "\n") print(name_1) # It returns a new copy. Hence fresh copy is not affected print("id of the community_1 variable ", id(name_1), "\n") print(name_2) # Both the variables points to same memory reference. Hence doing any operation also reflect to new reference. print("id of the community_2 variable ", id(name_2), "\n") """ output: ['co-learning', 'lounge', 'is', 'the', 'best'] id of the community variable 139779951152016 ['co-learning', 'lounge', 'is', 'the', 'best', 'community'] id of the community_1 variable 139779939162384 ['co-learning', 'lounge', 'is', 'the', 'best'] id of the community_2 variable 139779951152016 """
Counting the number of values in the list using the count() function
We can count the occurrence of any value in the list by using the count function. It takes one argument (value).
desc = "Co-Learning Lounge is the name of the community and it is best community among all" desc_list = desc.split(" ") print(desc_list) print(desc_list.count("community")) """ output: ['Co-Learning', 'Lounge', 'is', 'the', 'name', 'of', 'the', 'community', 'and', 'it', 'is', 'best', 'community', 'among', 'all'] 2 """
Removing Elements from the List
Using remove()
method
Elements can be removed from the List by using the built-in remove() function, but an Error arises if the element doesn’t exist in the set. The remove() method only removes one element at a time; to remove a range of elements, the iterator is used.
The remove() method removes the specified item only.
Note — Remove method in List will only remove the first occurrence of the searched element.
# Python program to demonstrate # Removal of elements in a List # Creating a List List = [1, 2, 3, 4, 5, 6, 5, 7, 8, 9, 7, "ten", [11, 12]] print("Intial List: ") print(List) # Removing elements from List # using Remove() method List.remove(5) print("\nList after removing 5 '(the first occurance of 5 from our list)': " ) print(List) List.remove("ten") print("\nList after removal of 'ten': ") print(List) """ output: Intial List: [1, 2, 3, 4, 5, 6, 5, 7, 8, 9, 7, 'ten', [11, 12]] List after removing 5 '(the first occurance of 5 from our list)': [1, 2, 3, 4, 6, 5, 7, 8, 9, 7, 'ten', [11, 12]] List after removal of 'ten': [1, 2, 3, 4, 6, 5, 7, 8, 9, 7, [11, 12]] """
We can loop through the list to delete multiple elements from a list.
Let’s try to delete some values from a range!
List = [1, 0, 2, 3, 4, 5, 6, 5, 7, 8, 9, 7, "ten", [11, 12]] print("Intial List: ") print(List) # Removing elements from List # using iterator method for i in range(1, 7): List.remove(i) print("\nList after removing a range of elements: ") print(List) """ output: Intial List: [1, 0, 2, 3, 4, 5, 6, 5, 7, 8, 9, 7, 'ten', [11, 12]] List after removing a range of elements: [0, 5, 7, 8, 9, 7, 'ten', [11, 12]] """
Let’s try another example!
a = ["my", "CLL", "great", "is", "USA", "helping", "me", "random", "with", "Python!"] for i in ["my", "great", "USA", "random"]: a.remove(i) print(a) """ output: ['CLL', 'is', 'helping', 'me', 'with', 'Python'] """
There is one in-built function clear()
to delete all elements at once.
Let’s try!
# Clearing all the values of the list List.clear() print("\nList after removing all the values: ") print(List) """ output: List after removing all the values: [] """
Using pop() method
The pop () function can also be used to remove and return an element from the set, but by default, it removes only the last element of the set. To remove the element from a specific position of the List, the index of the element is passed as an argument to the pop() method.
List = [1,2,3,4,5] # Removing element from the # Set using the pop() method # If index is not mentioned that it will pop last element List.pop() print("\nList after popping an element: ") print(List) # Removing element at a # specific location from the # Set using the pop() method List.pop(2) popped_value = List.pop(2) print("\nList after popping a specific element: ") print(List) print(popped_value) """ output: List after popping an element: [1, 2, 3, 4] List after popping a specific element: [1, 2] 4 """
the pop() method can delete value at any particular index. In remove, we could delete the value by entering the value itself as an argument, but with pop(), we can enter the index to delete the values.
List.pop(20) ## When the index value is not part of list print(List) """ output: --------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-16-22b95d028801> in <module> ----> 1 List.pop(20) ## When the index value is not part of list 2 print(List) IndexError: pop index out of range """
Using del method
just like the del keyword can be used to delete the entire list, it can also be used to delete certain values.
Let’s see how!
List = [1, 2, 3, 4, 5, 6, 7] ## Del 3 --> 2 ## Del 5 --> 4 del List[2] print("\nList after removing second index value ") print(List) del List[4] print("\nList after removing second index value ") print(List) del List[3:6] print("\nList after removing from third index to fifth (6 - 1) value ") print(List) ## Entire list can be deleted too del List print(List) ## Error because List variable is just deleted """ output: List after removing second index value [1, 2, 4, 5, 6, 7] List after removing second index value [1, 2, 4, 5, 7] List after removing from third index to fifth (6 - 1) value [1, 2, 4] --------------------------------------------------------------------------- NameError Traceback (most recent call last) <ipython-input-17-c604b03b77fe> in <module> 18 ## Entire list can be deleted too 19 del List ---> 20 print(List) 21 22 ## Error because List variable is just deleted NameError: name 'List' is not defined """
Difference between .remove(), .pop() and del
The pop() returns deleted value, whereas the remove() method doesn’t return any value. The del keyword can delete a single value from a list or the entire list at a time.
pop() takes the index as the argument, while remove takes the value itself as the argument.
List Methods
Python has a set of built-in methods that you can use on lists.
Method Description
append(): Adds an element at the end of the list
clear(): Removes all the elements from the list
copy(): Returns a copy of the list
count(): Returns the number of elements with the specified value
extend(): Add the elements of a list (or any iterable), to the end of the current list
index(): Returns the index of the first element with the specified value
insert(): Adds an element at the specified position
pop(): Removes the element at the specified position
remove(): Removes the item with the specified value
reverse(): Reverses the order of the list
sort(): Sorts the list
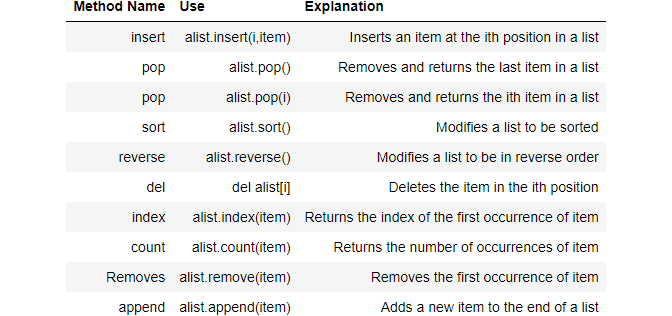
We have tried all methods and operations in lists. Lists are essential data types; hence, these operations will be used in logical problem-solving.
Please try these methods once, as most of these respond in a similar way in other datatypes as well. 🙂