Operations on List Datatype in Python
The list is a very powerful datatype, and it is vital to understand its features and powers to optimize our codes. Lists can contain different data types, and we will use lists very frequently as this is the most used data type.
We will try to access size, value replacing, slicing, and aggregation operations on Lists. We have also worked on such operations in strings, but that won’t be required for now.
Let’s try some examples. First, let’s try to find the size of the lists!
Knowing the size of the List
Just like we used the len()
function in strings to get the length of strings, similarly, we can use the same function to get the length of Lists.
Let’s try it once!
# Creating a List List1 = [] #No items in the list; hence, the length is 0. print(len(List1)) # Creating a List of numbers List2 = [10, 20, 14] print(len(List2)) """ output: 0 3 """
Accessing elements from the List
To access the list items refer to the index number. Use the index operator [ ] to access an item in a list. The index value should always be an integer.
# Python program to demonstrate # accessing of an element from the list # Creating a List with # the use of multiple values List = ["Co-", "Learning", "lounge"] # accessing an element from the # list using index number print("Accessing an element from the list") print(List[0]) print(List[2]) """ output: Accessing an element from the list Co- lounge """
Nested lists are accessed using nested indexing.
# Creating a Multi-Dimensional List # (By Nesting a list inside a List) List = [['co-', 'learning'] , ['lounge']] # accessing an element from the # Multi-Dimensional List using # index number print("Accessing a element \"lounge\"") print(List[0]) ## First accessing the first element i.e index 0 print(List[0][1]) ## Now from the first element result we need to access second element i.e index 1 """ output: Accessing an element "lounge" ['co-', 'learning'] learning """
You can refer to this picture to learn about the indexing of the previous example!
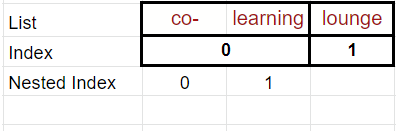
Replacing values in the list
We can easily replace the values in Lists. We have to access the index and assign a new value.
Let’s see what this means!
List = [1,2,3,4] ## Replacing 2 with 22 List[1] = 22 print(List) """ output: [1, 22, 3, 4] """
Negative indexing
In Python, negative sequence indexes represent positions from the end of the array. Instead of having to compute the offset as in List[len(List)-3], it is enough to just write List[-3]. Negative indexing means beginning from the end, -1 refers to the last item, -2 refers to the second-last item, etc.
Negative indexing works similarly to what we saw in strings.
List = [1, 2, 'co-', 4, 'learning', 6, 'lounge'] # accessing an element using # negative indexing print("Accessing element using negative indexing") # print the last element of the list print(List[-1]) print(List[6]) ## At this point index 6 is similar to index -1 # print the third last element of the list print(List[-3]) """ output: Accessing elements using negative indexing lounge lounge learning """
This is how it works!

Slicing of a List
In Python List, there are multiple ways to print the whole List with all the elements, but we use the Slice operation to print a specific range of elements from the list. Slice operation is performed on Lists using a colon(:), just like we do in strings.
- To print elements from the beginning to a range, use [: Index]
- To print elements from end-use [:-Index]
- To print elements from a specific Index till the end, use [Index:]
- To print elements within a range, use [Start Index: End Index]
- To print the whole List using the slicing operation, use [:]. Further, to print the whole List in reverse order, use [::-1].
Note — To print elements of the List from the rear end, use Negative Indexes.
name = "Co-Learning Lounge" print(list(name)) """ output: ['C', 'o', '-', 'L', 'e', 'a', 'r', 'n', 'i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] """
# Python program to demonstrate # Removal of elements in a List # Creating a List # List = ['c','o','-','l','e','a','r','n','i','n','g','l','o','u','n','g','e'] community_name = "Co-Learning Lounge" List = list(community_name) print("Intial List: ") print(List) # Print elements of a range # using Slice operation Sliced_List = List[3:8:1] # Starting Index is 3 and Ending index is 8-1 i.e 7 # So it will print from the 3rd to the 7th index print("\nSlicing elements in a range 3-8: ") print(Sliced_List) # Print elements of a range # using Slice operation Sliced_List = List[::2] # Printing every 2nd element print("\nPrinting every 2nd element: ") print(Sliced_List) # Print elements of a range # using Slice operation Sliced_List = List[:10:2] # Printing every 2nd element till 10th char print("\nPrinting every 2nd element till 10th char: ") print(Sliced_List) # Print elements of a range # using Slice operation Sliced_List = List[10::2] # Printing every 2nd element from 10th char print("\nPrinting every 2nd element from 10th char: ") print(Sliced_List) # Print elements from a # pre-defined index to end Sliced_List = List[5:] print("\nElements sliced from 5th " "element till the end: ") print(Sliced_List) # Print elements from a # Start to a pre-defined point Sliced_List = List[:7] print("\nElements sliced till 6th (7-1): ") print(Sliced_List) # Printing elements from # beginning till the end Sliced_List = List[:] print("\nPrinting all elements using slice operation: ") print(Sliced_List) """ output: Initial List: ['C', 'o', '-', 'L', 'e', 'a', 'r', 'n', 'i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] Slicing elements in a range of 3-8: ['L', 'e', 'a', 'r', 'n'] Printing every 2nd element: ['C', '-', 'e', 'r', 'i', 'g', 'L', 'u', 'g'] Printing every 2nd element till the 10th char: ['C', '-', 'e', 'r', 'i'] Printing every 2nd element from 10th char: ['g', 'L', 'u', 'g'] Elements sliced from 5th element till the end: ['a', 'r', 'n', 'i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] Elements sliced till 6th (7-1): ['C', 'o', '-', 'L', 'e', 'a', 'r'] Printing all elements using slice operation: ['C', 'o', '-', 'L', 'e', 'a', 'r', 'n', 'i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] """
We have also used the same kind of operations in stings as well. You can refer to the visuals to understand how it works in previous examples.
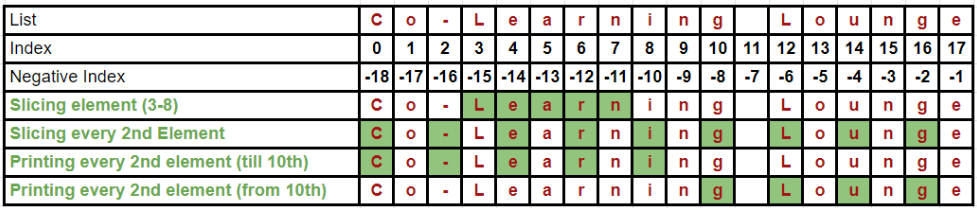
Negative index List slicing
Negative indexing is also some kind of slicing; it’s just that the indexing starts backward.
Let’s try some more examples!
# Creating a List # List = ['c','o','-','l','e','a','r','n','i','n','g','l','o','u','n','g','e'] community_name = "Co-Learning Lounge" List = list(community_name) print("Intial List: ") print(List) # Print elements using a # negative index Sliced_List = List[-5:-2] print("\nElements sliced from last 5 to last 3 (-2 - 1)") print(Sliced_List) # Print elements using a negative index # From start till last 5th char Sliced_List = List[:-5] print("\nElements sliced from start till last 6 (-5 - 1)") print(Sliced_List) # Print elements using a negative index # From last 10 char till end Sliced_List = List[-10:] print("\nElements sliced from last 10 char till end") print(Sliced_List) # Printing elements in reverse # using Slice operation Sliced_List = List[::-1] print("\nPrinting List in reverse: ") print(Sliced_List) """ output: Intial List: ['C', 'o', '-', 'L', 'e', 'a', 'r', 'n', 'i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] Elements sliced from last 5 to last 3 (-2 - 1) ['o', 'u', 'n'] Elements sliced from start till last 6 (-5 - 1) ['C', 'o', '-', 'L', 'e', 'a', 'r', 'n', 'i', 'n', 'g', ' ', 'L'] Elements sliced from last 10 char till end ['i', 'n', 'g', ' ', 'L', 'o', 'u', 'n', 'g', 'e'] Printing List in reverse: ['e', 'g', 'n', 'u', 'o', 'L', ' ', 'g', 'n', 'i', 'n', 'r', 'a', 'e', 'L', '-', 'o', 'C'] """
Membership operation:
Membership operators in Python check for membership of elements in a list or some other datatypes.
It checks if one element is present in the original list. There are two membership operators in
and not in
Let’s try to see some examples!
#We have one long string, let's start by spliting it desc = "Co-Learning Lounge is the name of the community and it is best community of all" desc_list = desc.split(" ") print(desc_list) """ output: ['Co-Learning', 'Lounge', 'is', 'the', 'name', 'of', 'the', 'community', 'and', 'it', 'is', 'best', 'community', 'among', 'all']
Let’s check if the community is present in our List with the membership operator!
'community' in desc_list """ output: True """
As seen in the above example, the membership operators result in True
and False
.
Let’s check some other words!
'Learning' in desc_list """ output: False """
Values that we are testing should be an exact match only then True
return.
For e.g, We have ‘Lounge’ in our list and will check for ‘lounge’.
'lounge' in desc_list """ output: False """
Comparison Operators
Comparison Operators compare the values on both sides and return True
or False
. We have tried these operators in numeric data types as well. These are also called relational operators.
Let’s try with lists!
X = [1, 2] Y = [1] X == Y """ output: False """
Y.append(2) X == Y """ output: True """
Identity Operators
Identity operators check if two objects are similar. The critical distinction is it checks the similarity, not equality, of two things. is
and is not
are the operators.
See the following example to understand.
X = ["Co-Learning", "Lounge"] Y = ["Co-Learning", "Lounge"] X is Y """ output: False """
In the above example, though X and Y are equal, it returns false because X and Y are equal but not similar; these are two different objects.
Let’s see an example of similar objects!
X = ["Co-Learning", "Lounge"] Y = ["Co-Learning", "Lounge"] Z = X print(X is Y) #Comparing with Identity operator print(X == Y) #Checking equal values with comparison operator print(X is Z) #Comparing with Identity operator # It return true because z and X are same objects. """ output: False True True """
X = ["Co-Learning", "Lounge"] X is ["Co-Learning", "Lounge"] # It return False """ output: False """
Arithmetic Operators
Arithmetic operators function in a similar manner as they do with datatypes.
See the following example to understand
a = ["Colearning", "Lounge"] b = ["helps", "to learn"] c = ["with", "interesting", "references"] print(a+b+c) """ output: ['Colearning', 'Lounge', 'helps', 'to learn', 'with', 'interesting', 'references'] """
Aggregation on the List
We can also perform aggregations on the lists.
Let’s try to find the Sum, minimum value, and maximum value from a list.
List = [6, -1, 7, 1, 3, 9, 0, -19, 2, 8, 4, 10, 27, 219, 1910] print("Sum of all the value is: ", sum(List)) print("max of all the value is: ", max(List)) print("max of all the value is: ", min(List)) """ output: Sum of all the value is: 2186 max of all the value is: 1910 max of all the value is: -19 """
We can also split the list with the split function and find the longest and shortest string.
Let’s try this out!
desc = "Co-Learning Lounge is the name of the community and it is best community among all" desc_list = desc.split(" ") print(desc_list) print(max(desc_list, key=len)) print(min(desc_list, key=len)) """ output: ['Co-Learning', 'Lounge', 'is', 'the', 'name', 'of', 'the', 'community', 'and', 'it', 'is', 'best', 'community', 'among', 'all'] Co-Learning is """
This blog has covered the basic operations of lists and aggregations. We recommend you try these operations and string methods in a rough python notebook.
It is not essential to remember all the methods because one can find these with one click on the internet. The important thing is to have tried these operations, so we know how we try each of these and how Lists respond. It is like understanding the powers of Lists to use those to finish the game quickly and efficiently! 🙂