Working with Strings in Python
Strings are one of the most important data types in Python. We are going to find ourselves dealing with strings very frequently. So far, we know how to create a string in Python. Now we are going to take a leap and learn how to access string characters, index in strings, access a part of the string, deleting and update strings.
It is a crucial step to mastering strings. This will help us later in breaking logical problems to form step-by-step logic.
Length of Strings
We can find the length of any strings in python using the len function.
Let’s try it once!
len("Hello") """ output: 5 """
Let’s try another example!
Community = "Co-Learning Lounge" len(Community) """ output: 18 """
Accessing characters in Python
In Python, individual characters of a String can be accessed using the Indexing method. You can access the character at the Nth position by putting the number N in between two square brackets ([ and ]) immediately after the string
Indexing allows negative address references to access characters from the back of the String, e.g., -1 refers to the last character, -2 refers to the second last character, and so on.
Notice that you don’t have to close each line with a quotation mark. Normally, Python would get to the end of the first line and complain that you didn’t close the string with a matching double quote. With a backslash at the end, however, you can keep writing the same string on the next line.
When you print() a multiline string that is broken up by backslashes, the output is displayed on a single line.
Understanding indexing is a very important concept for accessing characters in the string.
Let’s understand the concept of indexing by looking at one string and its indexes. Have a look at the following picture!

The index(if we start from left to right) starts from 0. Hence the first character of our string, “Co-Learning Lounge,” is at index 0, and the last character is at index 17.
Let’s see how to access the first and last characters of this!
# Python Program to Access # characters of String Community = "Co-Learning Lounge" Community[0] #We are trying to access the first character of out string """ output: 'C' """
# Python Program to Access # characters of String Community = "Co-Learning Lounge" Community[17] #We are trying to access the last character of out string """ output: 'e' """
Similarly, we can access any character of any string by putting the index in square brackets.
There is one catch here, in most cases, we are going to deal with longer strings, and it would be a waste of time to count the index to find the last or second last characters.
In such cases, we will use negative indexing. It means the last index is -1, and -2 is the second last index. (As shown in the above picture)
# Python Program to Access # characters of String Community = "Co-Learning Lounge" Community[-1] #We are trying to access the last character of out string (with negative indexing) """ output: 'e' """
If we try to access the index out of the range, it will cause an IndexError
.
Let’s see another example. So we know “Co-Learning Lounge” has 17 indexes.
# Python Program to Access # characters of String Community = "Co-Learning Lounge" Community[18] #We are trying to access the out of index """ output: --------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-4-55d74e126864> in <module> 4 Community = "Co-Learning Lounge" 5 ----> 6 Community[18] #We are trying to access the out of index IndexError: string index out of range """
String1 = "Co-Learning Lounge" print(String1[-20]) """ output: --------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-49-27bce0b80a0a> in <module> 1 String1 = "Co-Learning Lounge" ----> 2 print(String1[-20]) IndexError: string index out of range """
Only Integers are allowed to be passed as an index, float, or other types, will cause a TypeError
.
print(String1[4.0]) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-50-66e51050fc05> in <module> ----> 1 print(String1[4.0]) TypeError: string indices must be integers """
print(String1["4"]) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-51-3ae74712976e> in <module> ----> 1 print(String1["4"]) TypeError: string indices must be integers """
print(String1[int(4.0)]) #in this example, we are converting float to int, and it works """ output: e """
String Slicing
We know how to access any individual character in Python but what if we need to access multiple characters at the same time? We use string slicing for that.
For e.g., if you have name stings that contain first name and last name and you want to access not just any individual character but the first name, in that case, you use slicing.
To access a range of characters in the String, the method of slicing is used. Slicing in a String is done using a Slicing operator (colon).
Suppose we have one string, “Co-Learning Lounge,” and we want to access Co-learning only. We will use the initial character index and the last character index divided by a colon.
Let’s try it out!
String1 = "Co-Learning Lounge" print(String1[0:10]) ## [Start : Stop] # To access Co-Learning, we start from "C" and go to "L". # Index of "C" is 0 # Index of "L" is 10 """ output: Co-Learnin """
If we see in the above example, “L” is not included in the output? Wondering why?
By default, the stop index is excluded. To include the same, i.e., “L”, we have to go till “L” + 1 i.e., 11th index.
let’s try again!
String1 = "Co-Learning Lounge" print(String1[0:11]) #To access Co-Learning, we start from "C" and go to "L". #Index of "C" is 0 #Index of "L" is 10 """ output: Co-Learning """
See the picture to understand the index and substring of the previous example.
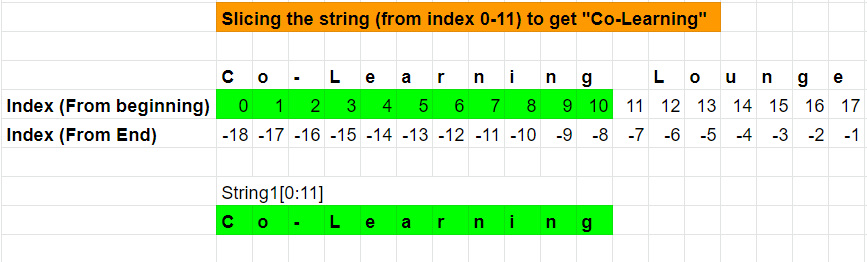
This is called String slicing because we have sliced the string to get the desired sub-string.
You can try achieving the same output with negative indexing (from end). We will cover this in examples.
# Python Program to # demonstrate String slicing # Creating a String String1 = "Co-Learning Lounge" print("Initial String: ") print(String1) # Printing 3rd to 12th character print("\nSlicing characters from 3-12: ") print(String1[3:12]) ## [start : end] Goes till (end - 1) """ output: Initial String: Co-Learning Lounge Slicing characters from 3-12: Learning """
Let’s discuss another important point here in slicing. So when we mention values in brackets [a:b]
, how does python extract the substring?
By default, the step value is 1.
Wondering what a step value is?
Let’s break it down!
in the last example, we are trying to access the substring from [3:12]
from our original string.
Python saves index three first! — ‘L’ after that, because the step value is 1, so python adds 1 to the last index. — 3+1 = 4 Python save index four! — ‘e’ Again step value is 1, so python adds 1 to the last index. — 4+1 = 5 Python save index five! — ‘a’
This is how Python saves the values of each step!
Now, the question is, can we change the step value from the default one?
The answer is yes!
Let me show you.
# Creating a string! Magic = "PKYXTEHIOFN" #Now in this string, unlike previous ones, we want to access every 2nd character. x = Magic[0:11:2] # 0 is initial index # 11 is final index # 2 is step value (changed from default 1) print(x) """ output: PYTHON """
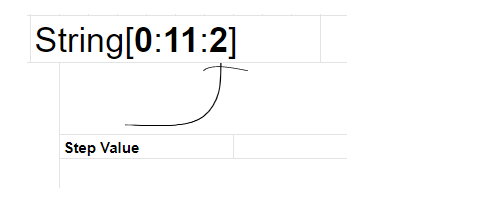
Let’s break it down with one picture.
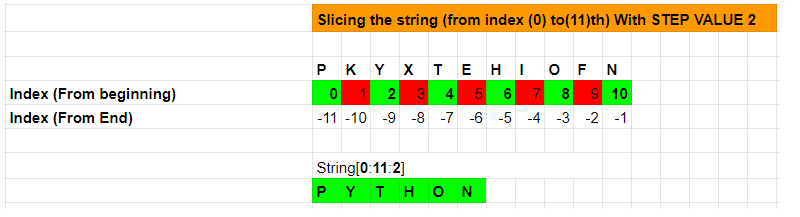
In the above example, Python starts at index 0 and goes to 11 with a step value of 2.
First it saves index 0 ==”P” Step value = +2 so it will check (0+2) index now. Second it saves index 2 = “Y” Step value = +2 so it will check (2+2)index now.
This is how the substring is sliced. If there isn’t any step value, then the default step value will be 1.
Let’s try accessing the substring from 3rd character to 4th last character.
# Printing characters between # 3rd and 4th last character String1 = "Co-Learning Lounge" print("Slicing characters between " + "3rd and 4th last character: ") print(String1[3:-4]) ## end - 1 --> -4 - 1 --> -5 """ output: Slicing characters between 3rd and 4th last character: Learning Lo """
Let’s see what did we do in the previous example:
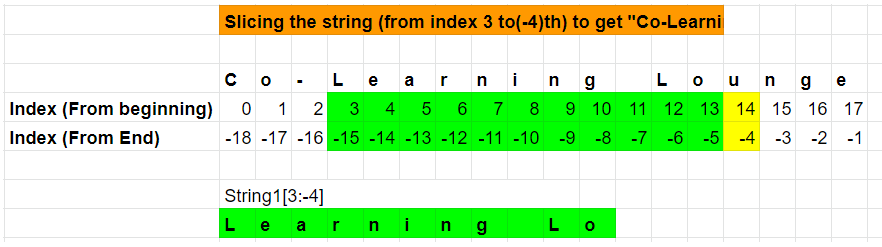
We can see -4th character is not included. If we want to include 4th last character, we need to go to the -3 index.
# Printing 4th to last character print("Slicing characters from 4-till end: ") print(String1[4:]) """ output: Slicing characters from 4-till end: earning Lounge """
If we want to start slicing the string from the very beginning (Index = 0), we don’t need to mention any index on the left side of the colon.
This means [0: n]
and [ : n]
is the same.
See the following example.
# If you omit the first index in a slice, Python assumes you want to start at index 0 print("Slicing characters from Start-10: ") print(String1[:10]) print(String1[0:10]) """ output: Slicing characters from Start-10: Co-Learnin Co-Learnin """
Similarly, if we want to access till the last character, we don’t need to mention that also.
This means [n:last]
or [n: ]
is the same.
Let’s see the example!
# If you omit both the first and second numbers in a slice, you get a string that starts with the character with index 0 and ends with the last character. print("\nOmitting both numbers in a slice returns the entire string") print(String1[:]) #We we are trying to slice from index 0 to last index (Basically the entire string) print(String1) """ output: Omitting both numbers in a slice returns the entire string Co-Learning Lounge Co-Learning Lounge """
Easy and exciting so far, right?
What if we try to access one index that doesn’t exist? For example, “Co-Learning Lounge” has an index of 17. What if we try to access index 20?
Will it throw an error?
The answer is NO! Let’s see how?
## Interesting Scenarios """ In the below slicing we are trying to access from the start till 20th character but we only have characters till 17. Here Python won't throw `IndexError`. Instead, any non-existent indices are ignored and the entire string "Co-learning lounge" is returned. """ print(String1[:20]) print(String1[5:20]) """ output: Co-Learning Lounge arning Lounge """
The above code doesn’t throw an error. Instead, it returns till the last index. 🙂
What if instead of trying to access from 0–20, we try to access from 18 to 20 index? (Both don’t exist in our string)
Will it run, or we encounter an error? Let’s find out!
""" The second shows what happens when you try to get a slice where the entire range is out of bounds. String1[25:30] attempts to get the 25th and 30th characters, which don't exist. Instead of raising an error, the empty string "" is returned. """ print(String1[25:30]) """ output: """
The above code doesn’t throw an error. It returns an empty string instead.
Cool, right?
Let’s try to access some more substrings, but this time with negative indexing.
## Using negative numbers in slices print(String1[-9:-6]) """ output: ng """
See the visual to understand what Python did in the last code.
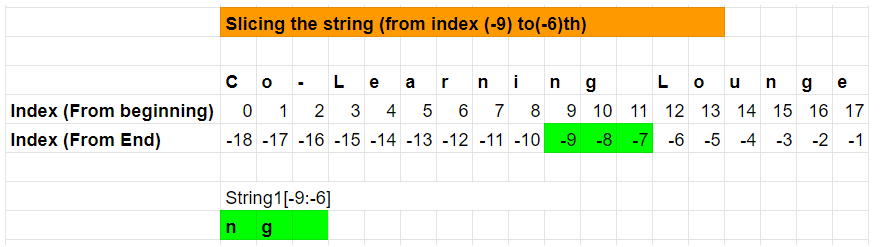
print(String1[-9:-1]) """ output: ng loung """
If you have noticed one thing so far, we always go left to right in all our codes.
What if we try right to the left?
Let’s try!
# The logical choice for that right most boundary would seem to be the number 0, but that doesn't work print(String1[-9:0]) """ output: """
Accessing from right to left returns an empty string even if characters exist on these indexes. The reason is the logical problem.
See, when we are trying to access String[1:5]
, python starts at index 1 and then increments +1 on each step and breaks out when it reaches to 5th index (hence 5th index is not included in our outputs).
But when we try to go say String[5:1]
or String[-6:-9]
, the problem is that Python starts at 5, then it tries to increment +1 this way, it will never reach 1 index, and because of this wrong logic, it returns the empty string.
Same goes with String[-6:-9]
, It starts at -6, and then it increments +1, so -6 will never reach -9 and hence again empty string.
language = "Co-Learning Lounge" print(language[-1:-7:-1]) """ output: egnuoL """
To change this default incremental +1, we can use -1 at the end like the previous example.
Try once to access the right to the left characters (reverse strings). 🙂
We can also change this incremental to any value. Suppose we want to access every second character in the range; we can use +2 there.
Let’s see this!
alphabets = "23456789" print(alphabets[0:7:2]) #This start from index 0 and goes till index 7 #instead of getting every character, now it will take every second character """ output: 2468 """
Deleting/Updating from a String
In Python, the Updation or deleting of characters from a String is not allowed. This will cause an error because item assignment or item deletion from a String is not supported. However, deletion of the entire String is possible with a built-in del keyword. This is because Strings are immutable, hence elements of a String cannot be changed once assigned. Only new strings can be reassigned to the same name.
# Python Program to Update # character of a String String1 = "Hello, I'm a part of Co-Learning Lounge's community" print("Initial String: ") print(String1) # Updating a character # of the String String1[2] = 'p' print("\nUpdating character at 2nd Index: ") print(String1) """ output: Initial String: Hello, I'm a part of Co-Learning Lounge's community --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-3-f51ab057a9c2> in <module> 8 # Updating a character 9 # of the String ---> 10 String1[2] = 'p' 11 print("\nUpdating character at 2nd Index: ") 12 print(String1) TypeError: 'str' object does not support item assignment """
We can see in the above example it is not possible to assign any character on any index because of the immutable property of the string.
If we want to change something, we have to assign an entirely new string.
String1[0:5] = 'Hey' print("\nReplacing the first word: ") print(String1) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-65-067f0283ad16> in <module>() ----> 1 String1[0:5] = 'Hey' 2 print("\nReplacing the first word: ") 3 print(String1) TypeError: 'str' object does not support item assignment """
We can’t add ‘Hey’ before our string. To do this, we have to assign a new string like the following example.
String1 = "Hey, I'm a part of Co-Learning Lounge's community"
alternatively, we can add “Hey” string to our sub-string like the next example.
String1 = "Hello, I'm a part of Co-Learning Lounge's community" String1 = "Hey" + String1[5:] print("Alternative way: ") print(String1) """ output: Alternative way: Hey, I'm a part of Co-Learning Lounge's community """
Updating Entire String:
As we have seen in the above examples, updating something in a string is not possible. Hence, we always have to assign a new strings.
# Python Program to Update # entire String String1 = "Hello, I'm a part of Co-Learning Lounge's community" print("Initial String: ") print(String1) # Updating a String String1 = "Hey, I am member of Co-Learning Lounge" print("\nUpdated String: ") print(String1) """ output: Initial String: Hello, I'm a part of Co-Learning Lounge's community Updated String: Hey, I am member of Co-Learning Lounge """
Deletion of a character:
As we have understood so far, strings are immutable, and we can’t update any particular character or simply we can’t make particular changes.
Similarly, we can’t delete one character as well. We can delete entire strings if we want but deleting particular characters doesn’t work in Python.
String1 = "Hello, I'm a learner" String1[2] """ output: 'l' """
# Python Program to Delete # characters from a String String1 = "Hello, I'm a learner" print("Initial String: ") print(String1) # Deleting a character # of the String del String1[2] print("\nDeleting character at 2nd Index: ") print(String1) """ output: Initial String: Hello, I'm a learner --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-7-e8f3af8a116c> in <module> 8 # Deleting a character 9 # of the String ---> 10 del String1[2] 11 print("\nDeleting character at 2nd Index: ") 12 print(String1) TypeError: 'str' object doesn't support item deletion """
del String1[2:5] print("\nDeleting character at 2nd to 5th Index: ") print(String1) """ output: --------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-8-5212244ae357> in <module> ----> 1 del String1[2:5] 2 print("\nDeleting character at 2nd to 5th Index: ") 3 print(String1) TypeError: 'str' object does not support item deletion """
Deleting Entire String:
Deletion of the entire string is possible with the use of the del keyword. Further, if we try to print the string, this will produce an error because the String is deleted and is unavailable to be printed.
# Python Program to Delete # entire String String1 = "Hello, I'm a learner" print("Initial String: ") print(String1) # Deleting a String # with the use of del del String1 print("\nDeleting entire String: ") print(String1) """ output: Initial String: Hello, I'm a learner Deleting entire String: --------------------------------------------------------------------------- NameError Traceback (most recent call last) <ipython-input-9-27108f753b91> in <module> 10 del String1 11 print("\nDeleting entire String: ") ---> 12 print(String1) NameError: name 'String1' is not defined """
This blog has covered basic operations in a string, including accessing strings, updating, and deleting.
There are many methods in strings that can make our lives easier. We are going to cover those in the next blog.