Print Function in Python
The most baby step while learning a programming language is to learn about the input and output statements. Output is not just for the functioning of our programs. It is essential for checking at various steps if our code is running fine. Also, it is used in debugging as well. If you have thousands of lines of code, it is complete nightmare to find any bug if things are not working as expected so developers check the outputs, step by step, and see which step is not giving output as expected to pinpoint the exact problem.
While learning Python, outputs are bliss. For beginners, it is something that is used if things are working.
In python, we use the print function as our output statement. In this blog, we are going to learn all about print statements along with the different print formatting techniques.
Let’s explore the output techniques!
"Hello World!" """ output: 'Hello World' """
In the above example, we are printing ‘Hello World. We can simply write any data type and the code editors will print it in output.
Most code editors will allow you to print this way. Let’s see another example!
"Hello World" "Hey, How you doin?" """ output: 'Hey, How you doin?' """
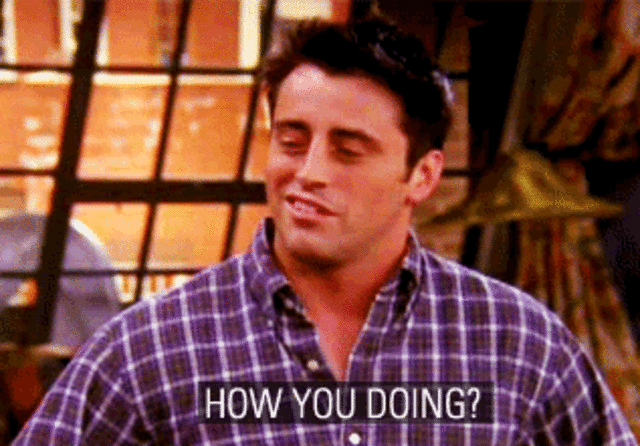
Did you see what happened here? We are trying to print ‘Hello World’ and ‘Hey, how you doin?’.
It only prints the second statement!
This way we can only print the latest string. As in the above example, the latest is the second statement, hence it printed only the second.
Let’s resolve it!
print("Hello World!") print("Hey, How you doin?") """ output: Hello World Hey, How you doin? """
In the above example, we are using print in the first statement and second statements.
print( ) is a function that is used to print the output. In parentheses, pass the data that should be printed. Remember, it is printed with a small p. 🙂
Let’s try to do some more printing!
print("Hello World!", "Hey, How you doin?") """ output: Hello World Hey, How you doin? """
We can pass multiple different values in print separated by a comma and it will print everything separated by a space. In the previous code, we tried the same.
print(value 1, value2, value3………..)
Syntax :
print() seems like a simple function but it has more values than that. Let’s try to know more about the print function and its feature. You will be amazed to know about it.
This is what the default print function looks like.
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
You can call the help function to know more about any function. Let’s try that.
help(print) """ output: Help on built-in function print in module builtins: print(...) print(value, ..., sep=' ', end='\n', file=sys.stdout, flush=False) Prints the values to a stream, or to sys.stdout by default. Optional keyword arguments: file: a file-like object (stream); defaults to the current sys.stdout. sep: string inserted between values, default a space. end: string appended after the last value, default a newline. flush: whether to forcibly flush the stream. """
We can see there are some things alreadyinbuilt and default in print(). If we see the above results, the first is the value (something that we want to print), the second is sep = “ “(separator- which separates multiple values, default is space), and the third is ending= ‘\n’ (NEW Line Character, to take us to the next line.
These things are called parameters. Let’s understand the parameters one by one with some examples.
Parameters :
When we are printing even a simple statement there is a lot that goes within the print function. Let’s break it down.
print(*objects, sep=’ ‘, end=’\n’, file=sys.stdout, flush=False)
file: a file-like object (stream); defaults to the current sys.stdout.
sep: string inserted between values, default a space.
end: string appended after the last value, default a newline.
flush: whether to forcibly flush the stream.
Let’s try some examples!
print(2) """ output: 2 """
a = 2 print(a) """ output: 2 """
a = 2 b = 3 print(a, b) """ output: 2 3 """
In the above example, we are printing two variables in one print. This is possible and there is default space between them. There is a default space separator.
Let’s try another one!
a = 2 b = 3 print(a, b, "Hello World") #The default space will be added regardless of data type. """ output: 2 3 Hello World """
print("Hey today we will learn about print function") """ output: Hey today we will learn about print function """
print("Sundar","Pichai",sep=" ") """ output: Sundar Pichai """
Did you see what is done in the above example?
We are printing two values which are “Sundar” and “Pichai”. There will a default space between both strings as there is a default space. We tried to specify it as sep = “”, and it works the same.
We can use any other separator as well if we don’t want the default space.
Let’s try one example:
print("Sundar", "Pichai", sep="_*") """ output: Sundar_*Pichai """
In the above code, we have changed the separator from “ ” to “_*” and it is printed instead of space in the output.
Cool, right?
Let’s see one more cool feature.
As we know now that a default space is inbuilt into the print function which can be changed to whatever character we want. There is a default NEWLINE (\n) character also in the print function.
Let’s check!
print("Hello World") print("How are you?") """ output: Hello World How are you? """
We can see, when we wrote to print functions, both outputs are in a different line. Why don’t these appear in the same line?
The reason is the new line character we talked about. When we write the print function, the default newline character takes us to a new line. It is similar to hitting enter on your keyword once you write something.
One example to x-ray this one-
print("Hello World") print() print("How are you?") """ output: Hello World How are you? """
What happened in the above code is after the first print is executed, by default it goes to the new line. The new line doesn’t have anything to print. Still, because of the print() function’s inbuilt new line character, it goes to the third line and the third print is executed.
That’s why we see one empty line.
Just like we changed the default space previously by specifying any other character, the same way we can change the default new line by specifying something.
Let’s see one more example!
print("Hello World", end="_") print() print("How are you?") """ output: Hello World_ How are you? """
In the above example, we are specifying _ with end = “_”. It prints the _ at the end and doesn’t go to the second line. It prints the second print function, which is empty, in the same first line.
The second function doesn’t have a specific end character specified, so the default is a new line, and hence the ‘How are you?’ is printed in a new line.
Let’s print all three in a single line!
print("Hello World", end="_") print(end="*") print("How are you?") """ output: Hello World_*How are you? """
for i in [1,2,3,4,5]: print(i, end="\n") """ output: 1 2 3 4 5 """
This is the loop which is printing all outputs in new line because of the new line character.
We need not to understand for loop now, we are focusing on print.
Let’s specify another character instead if new line character.
for i in [1,2,3,4,5]: print(i,end="-") """ output: 1-2-3-4-5- """
print (1, 2, 3, 4, 5, sep = "-") """ output: 1-2-3-4-5 """
Print formatting
String formatting basically is designing our strings using formatting techniques. we’ll learn about the four major approaches to string formatting in Python.
Using the below variables let’s print the sentence :
“Albert Einstein was born in 1879”
name = "Albert Einstein" year = 1879
Default way
print(name, "was born in", year) """ output: Albert Einstein was born in 1879 """
Using str.format
Strings in Python can be formatted with the use of the format() method which is a very versatile and powerful tool for the formatting of Strings. It is defined using curly brackets: { }. We can refer to the substitutions of a variable by name and use them in the order we want.
print("{0} was born in {1}".format(name,year))
# Python Program for # Formatting of Strings # Default order String1 = "{} {} {}".format('Co-Learning Lounge', 'is the', 'community') print("Print String in default order: ") print(String1) # Positional Formatting String1 = "{1} {0} {2}".format('Co-Learning Lounge', 'is the', 'community') print("\nPrint String in Positional order: ") print(String1) # Keyword Formatting String1 = "{e} {m} {s}".format(s = 'Co-Learning Lounge', m = 'is the', e = 'community') print("\nPrint String in order of Keywords: ") print(String1) # Keyword Formatting String1 = "{commuity} {sent} {type}".format(community = 'Co-Learning Lounge', sent = 'is the', type = 'community') print("\nPrint String in order of Keywords: ") print(String1)
Integers such as Binary, hexadecimal, etc., and floats can be rounded or displayed in the exponent form with the use of format specifiers
# Formatting of Integers String1 = "{0:b}".format(16) print("\nBinary representation of 16 is ") print(String1) # Formatting of Floats String1 = "{0:e}".format(165.6458) print("\nExponent representation of 165.6458 is ") print(String1) # Rounding off Integers String1 = "{0:.2f}".format(1/6) print("\none-sixth is : ") print(String1)
A string can be left() or center(^) justified with the use of format specifiers, separated by a colon(:)
# String alignment String1 = "|{:<10}|{:^10}|{:>10}|".format('co','learning','lounge') print("\nLeft, center and right alignment with Formatting: ") print(String1) # To demonstrate aligning of spaces String1 = "\n{0:^16} was founded in {1:<4}!".format("co-learning Lounge", 2020) print(String1)
using f-Strings
It prefixes the string with an “f”, henceforth the name f string. Expressions inside the string are evaluated by string. The expressions inside the curly braces will be replaced with their values.
print(f"{name} was born in {year}")
Using % operator
If you are someone who is well versed in the print-style function in C, you will recognize it instantly. In python, strings have a built-in operation that can be accessed with the % operator.
We will be using %s,%d format specifier here to tell Python where to substitute the value of name and year, represented as a string and integer correspondingly. This operation is commonly known as string interpolation.
print("%s was born in %d "% (name,year) )
# Python Program for # Old Style Formatting # of Integers Integer1 = 12.3456789 print("Formatting in 3.2f format: ") print('The value of Integer1 is %3.2f' %Integer1) print("\nFormatting in 3.4f format: ") print('The value of Integer1 is %3.4f' %Integer1)
A print statement using the sys library
We can also use the sys library for printing the output
import sys sys.stdout.write('Hello World')
We are going to use the print function a lot now that we have connected to python. For beginner’s level, In pattern-related questions, one can practice using these default arguments of print statements to understand and get a good hands-on experience. 🙂